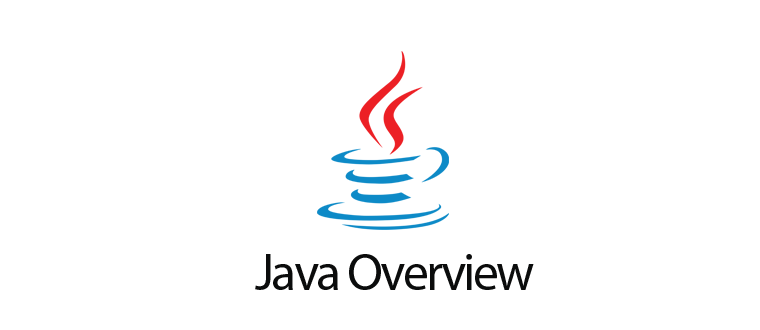
This is the first Java tutorial from a series of tutorials for beginners. If you want to learn Java in a simple way you are on the right place here. I wont go into great details in this tutorials. My goal is to teach you how to write Java code in no time! If you need…
Continue reading