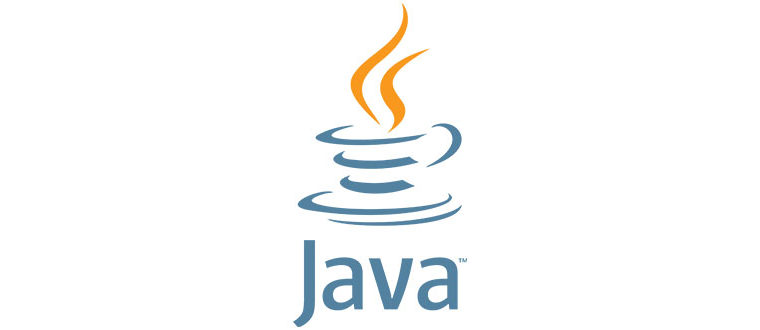
In this tutorial, we will be going through the installation process of Maven. If you are a WINDOWS user (if you are not, scroll down to view how to install Maven if you are a Linux or Mac user): Before proceeding with the next steps, make sure you have JDK installed on your system. If…
Continue reading