This example demonstrates the usage of inheritance in Java programming language
What is Inheritance
Inheritance is the OOP ability that allows Java classes to be derived from other classes. The parent class is called a superclass and the derivatives are called subclasses. Subclasses inherit fields and methods from their superclasses.
Inheritance is one of the four major concepts behind object-oriented programming (OOP). OOP questions are very common in job interviews, so you may expect questions about inheritance on your next Java job interview.
The “mother of all classes” in Java is the Object
class. Each and every class in Java inherits from Object
. At the top of the hierarchy, Object
is the most general of all classes. Classes near the bottom of the hierarchy provide more specialized behavior.
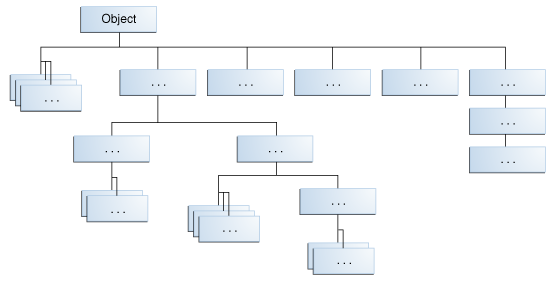
All Classes in the Java Platform are Descendants of Object (image courtesy Oracle)
Java has a single inheritance model, which means every class has one and only one direct superclass.
A subclass inherits all of the public and protected members of its parent, no matter what package the subclass is in. If the subclass is in the same package as its parent, it also inherits the package-private members of the parent. You can use the inherited members as is, replace them, hide them, or supplement them with new members:
- The inherited fields can be used directly, just like any other fields.
- You can declare a field in the subclass with the same name as the one in the superclass, thus hiding it (not recommended).
- You can declare new fields in the subclass that are not in the superclass.
- The inherited methods can be used directly as they are.
- You can write a new instance method in the subclass that has the same signature as the one in the superclass, thus overriding it.
- You can write a new static method in the subclass that has the same signature as the one in the superclass, thus hiding it.
- You can declare new methods in the subclass that are not in the superclass.
- You can write a subclass constructor that invokes the constructor of the superclass, either implicitly or by using the keyword
super
.
Inheritance is a powerful technique, which allows you to write clean and maintainable code. For example, let’s assume you have a superclass with multiple successors. It is much easier to change couple of lines of code in the superclass and by doing this to change the functionality of every inheritor instead of doing this in each end every subclass.
Java Inheritance Example
In the example below we create 3 classes. The superclass Point
represents a point with x and y coordinates in the 2-dimensional space.
package net.javatutorial; public class Point { // fields marking X and Y position of the point public int x; public int y; // one constructor public Point(int x, int y) { super(); this.x = x; this.y = y; } // getter and setter methods public int getX() { return x; } public void setX(int x) { this.x = x; } public int getY() { return y; } public void setY(int y) { this.y = y; } }
ColoredPoint
is a subclass which extends all the properties and methods of Point
and adds one additional field – colorName
. Note how this is done – we use the keyword extends to tell which class we want to derive from
package net.javatutorial; public class ColoredPoint extends Point { // new field added to store the color name public String colorName; public ColoredPoint(int x, int y, String colorName) { super(x, y); this.colorName = colorName; } public String getColorName() { return colorName; } public void setColorName(String colorName) { this.colorName = colorName; } }
And finally a program to test the inheritance. First we create a new Point
of type ColoredPoint
. Note the usage of instanceof keyword. This way we can check if an object is of certain type. Once we have determined point is of type ColoredPoint
we can explicitly type-cast by using:
ColoredPoint coloredPoint = (ColoredPoint)point;
now we can access the new property colorName
package net.javatutorial; public class InheritanceExample { public static void main(String[] args) { Point point = new ColoredPoint(2, 4, "red"); if (point instanceof ColoredPoint) { ColoredPoint coloredPoint = (ColoredPoint)point; System.out.println("the color of the point is: " + coloredPoint.getColorName()); System.out.println("with coordinates x=" + coloredPoint.getX() + " y=" + coloredPoint.getY()); } } }
Running the example above will produce following output
the color of the point is: red with coordinates x=2 y=4
References
Official Oracle Inheritance tutorial
In eclipse line 10 is giving an error. It shoud be coloredPoint.getColorName());
Thank you. I fixed it.