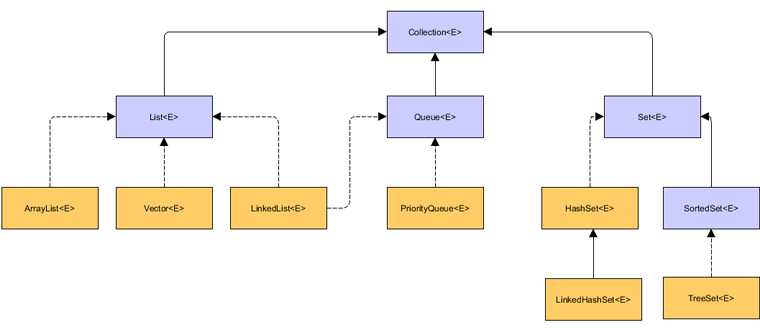
The “Collections” framework in Java came into action with the release of JDK 1.2 and was expanded quite a few times in Java 1.4 and Java 5 and then again in Java 6.
Continue readingThe “Collections” framework in Java came into action with the release of JDK 1.2 and was expanded quite a few times in Java 1.4 and Java 5 and then again in Java 6.
Continue readingJava offers you a variety of collection implementations to choose from. In general you will always look for the collection with the best performance for your programming task, which in most cases is ArrayList, HashSet or HashMap. But be aware, if you need some special features like sorting or ordering you may need to go for a special…
Continue readingJava ArrayList class is a resizable array that implements the List interface. It permits all elements, including null and also implements all optional list operations. Most operations that can be run on ArrayList such as size, isEmpty, get, set, iterator and listIterator are all constant time. However, the add operation’s complexity is O(n) time. Compared to LinkedList, the…
Continue readingLinkedList class in Java uses a doubly linked list to store elements and it also provides a linked-list data structure. It implements List, just like ArrayList class, and Deque interfaces. Just like arrays, Linked List is a linear data structure but unlike arrays, the elements in the linked list are linked together using pointers. There…
Continue readingCollections that use a hash table for storage are usually created by the Java HashSet class. As the name suggests, HashSet implements the Set interface and it also uses a hash table which is a HashMap instance. The order of the elements in HashSet is random. The null element is permitted by this class. In…
Continue readingJava TreeSet class is a NavigableSet implementation that is based on TreeMap. The elements are either ordered by a Comparator or simply by their natural ordering. In terms of complexity, this implementation provides log(n) time cost for all basic operations like add, remove, contains. What is important to know about TreeSet in Java TreeSet implements the…
Continue readingLinkedHashSet class in Java differs from HashSet as its implementation maintains a doubly-linked list across all elements. This linked list defines the iteration ordering which is the order in which the elements were inserted into the set. It is called an insertion-order. If an element is re-inserted into the set, the insertion order is not affected by…
Continue readingJava EnumSet class implements Set and uses it with enum types. EnumSet (as the name suggests) can contain only enum values and all the values belong to the same enum. In addition, EnumSet does not permit null values which means it throws a NullPointerException in attempt to add null values. It is not thread-safe which means if required,…
Continue readingJava 8 finally allows us, the programmers, to create thread-safe ConcurrentHashSet in Java. Before then, it was simply not possible. There were variations that tried to improvise the implementation of the above-mentioned class, one of which would be, using ConcurrentHashMap with a dummy value. However, as you might have guessed, all of the improvisations of…
Continue readingArrays’ items are stored as an ordered collection and we can access them by indices. HashMap class in Java on the other hand, stores items in a group pairs, key/value. They can be accessed by an index of another type. This class does not guarantee that there will be a constant order over time. HashMap provides…
Continue readingLinkedHashMap is a combination of hash table and linked list that implement the Map interface with predictable iteration order. The difference between HashMap and LinkedHashMap is that LinkedHashMap maintains a doubly-linked list which allows scanning through all of its entries back and forth. The order is maintained, meaning the order in which keys were inserted…
Continue readingTreeMap implements the Map interface and also NavigableMap along with the Abstract Class. The map is sorted according to the natural ordering of its keys or by a Comparator provided a the time of initialization. In terms of time complexity, this implementation provides log(n) cost for the containsKey, get, put and remove operations. It’s important…
Continue readingEnumMap class implements the Map class and enables the use of enum type keys. Enum maps are maintained in the natural order of their keys. It is important to note that null keys are not permitted. If attempt has been made for adding a null key, NullPointerException will be thrown. However, even though null keys are not allowed, null values are. Since all…
Continue readingWeakHashMap in Java implements the Map interface and represents a hash table that has weak keys. If a key is not in an ordinary use, the entry from the map will be automatically removed. This is what differentiates it from other Map implementations. Null and non-null values are supported and the performance is similar to HashMap class in and…
Continue readingIdentityHashMap implements the Map interface and two keys are considered equal when checked as k1==k2 (not by using equals method). This itself violates the Map‘s general contract, which means that IdentityHashMap is clearly not a general-purpose Map implementation. There are only so many cases where this class would be useful. IdentityHashMap permits both null values and the null key, alongside all optional map…
Continue readingEven though Map is implemented by many classes, many of them are not thread-safe or some of them are but not efficient. This is why ConcurrentMap was introduced in Java 1.5. It is thread-safe and efficient. Overridden default implementations: compute replaceAll forEach getOrDefault computerIfAbsent computerIfPresent ConcurrentMap consists of an array of nodes that are represented as table…
Continue readingHashtable implements a hash table (as the name suggests) and maps keys to values (like LinkedHashMap). Hashtable class allows non-null objects to be used as a key or as a value. Just like HashMap, Hashtable has two parameters that affect its performance: initial capacity and load factor. The capacity is the number of buckets in the hash…
Continue reading