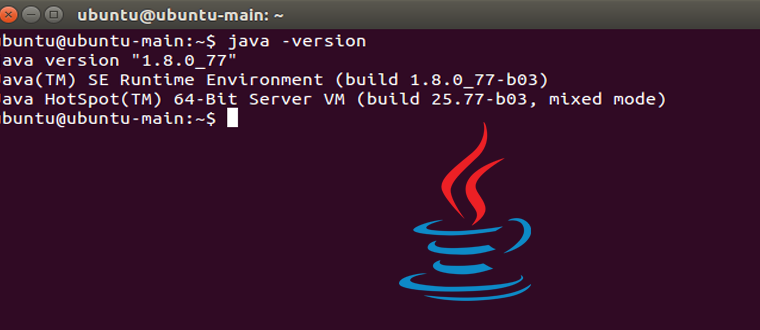
In this tutorial you will learn how to install latest Java 8 on Ubuntu or LinuxMint via PPA.
Continue readingIn this tutorial you will learn how to install latest Java 8 on Ubuntu or LinuxMint via PPA.
Continue readingIn this tutorial, we will be going through the installation process of Maven. If you are a WINDOWS user (if you are not, scroll down to view how to install Maven if you are a Linux or Mac user): Before proceeding with the next steps, make sure you have JDK installed on your system. If…
Continue readingSimply said, Maven profile is a set of configuration value which override default values. By using it, it allows you to create a custom build for different environments (Production/Development). Before we proceed with the tutorial’s content, it is assumed you have Maven installed. In case you don’t, follow this tutorial for a step-by-step guide. To…
Continue readingIf you’ve ever wondered whether you can upload your own libraries as a dependency using Maven, the answer is yes, you can. And it is really simple. It’s a 2-step process. Step 1 Navigate to your Maven project path in the command line and to upload a library, that’s the structure of the maven command:…
Continue readingWhat is software testing and why is it useful? Testing software in the simplest terms is to evaluate whether it meets all the requirements or not and to also inspect if there are any misbehaviors and bugs associated with its currents state. Testing in Java Java has multiple testing frameworks available, however, in this tutorial,…
Continue readingThis tutorial will cover how to run Unit tests using the Maven’s Surefire plugin. If you are not familiar with Unit Testing, you can follow this tutorial for a quick catch-up. In our Maven Project, we need the following mandatory dependencies: <dependencies> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.4.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>5.4.2</version> <scope>test</scope> </dependency> </dependencies>…
Continue readingWhat you will need. IDE or a text editor JDK 1.8 or above Maven What exactly is Maven’s multi-module? This multi-module project is built off of a POM aggregator that handles multiple submodules. Often the aggregator is located in the project’s root directory and must have packaging of type pom. The submodules are Maven projects…
Continue readingWe do not normally need additional dependencies when it comes to creating an executable jar. All we need to do is create a Maven Java project and have a minimum of 1 class alongside the main method. Please note that we assume you have Maven installed on your machine. In case you don’t, follow this tutorial…
Continue readingBefore proceeding with the next steps, make sure you have JDK and Maven installed on your system. If you don’t have JDK installed, click here. If you don’t have Maven installed, click here. 1. Generating a WAR file using Eclipse Step 1 – Open Eclipse and create a new Maven project (File->New->Other->Maven Project) Step 2…
Continue readingMemory can be described as an array of bytes where you can access each byte individually. In each byte or rather, location, in the memory, there is data that you can access, just like in arrays in Java. In 32-bit architectures, each memory “slot” contains 32 bits, or also called 1 word, or just 4…
Continue readingLet’s give an example first before going deeper into what Mutex is: Think of a queue. Doesn’t matter short or long. Now think of a truck that is selling tickets for an amusement park. One person at a time can buy a ticket. When the person has bought the ticket, it is time for the…
Continue readingSemaphore can be used to limit the amount of concurrent threads and essentially, this class maintains a set of permits. acquire() takes a permit from the semaphore and release() returns the permit back to the semaphore. In the event of absence of permits, acuire() will block until one is available Semaphore is used to control…
Continue readingStream API allows developers to take advatange of multi core architectures and improve the performance of Java program by creating parallel streams and making your program perform operations faster. There are two ways of creating a parallel stream: by using the parallelStream() method by using the parallel() method When you are using parallel streams, effectively…
Continue readingActive threads consume system resources, which can cause JVM creating too many threads which means that the system will quickly run out of memory. That’s the problem Thread Pool in Java helps solving. How Thread Pools work? Thread Pool reuses previously created threads for current tasks. That solves the problem of the need of too…
Continue readingThreadLocal is a class that provides thread local variable and is used to achieve thread safety. The data stored will be accessible only by a specific thread. ThreadLocal extends Object class and provides thread restriction which is a “part” from local variable. Creating a ThreadLocal variable ThreadLocal threadLocalExample = new ThreadLocal(); The instantiation of the…
Continue readingThe result of an asynchronous computation is called Future and more specifically, it has this name because it (the result) will be completed at a later point of time in the future. Future object is created when an asynchronous task has been created. There are many methods that are provided that help to retrieve the result (using get methods(which is…
Continue readingJava equals() method and the “==” operator are both used to compare objects for equality. However, they are doing the checking in a very different way, generating different results. The main difference between them is that “==” checks if both objects point to the same memory location and equals() evaluates to the comparison of the…
Continue readingJava 8 introduced Lambda Expressions and is one of the biggest, if not the biggest, feature that was introduced because Lambda expression makes functional programming possible and it makes your code way cleaner and overall simplifies the whole code implementation drastically. For example, when you have to work with anonymous class and that class is…
Continue readingJava 8 introduced Optional class which is used to manipulate data based on whether a value is present or absent. You can have the same functionality without using this class, however you would end up with way messier code. In other words, you will have less null checks and no NullPointerException’s. Let me give you…
Continue readingJava 11 introduced the HTTP Client which can be used to send requests over the network and retrieves their responses. HTTP Client replaces the HttpUrlConnection class as it is considered old and doesn’t support ease of use. HTTP Client API supports both HTTP/1.1 and HTTP/2. HttpClient is also immutable, meaning it can be used to…
Continue readingThis tutorial will help you understand how to perform basic database operations such as Create, Retrieve, Update and Delete (CRUD) using JDBC, which stands for Java Database Connectivity API. If you have used SQL before, you would be familiar with the INSERT, SELECT, UPDATE and DELETE statements. Effectively, CRUD and ISUD are the same. Before…
Continue readingEnum type is a special data type which holds different constants such as WHITE, BLACK, RED. The convention is that they should be named with upper case because, again, they are constants. In java, you define enum type by using the enum keyword. public enum Macronutrients { FATS, CARBOHYDRATES, PROTEIN } If you know all the…
Continue readingThe super class in Java java.lang.Object provides two important methods for comparing objects: equals() and hashcode(). These methods are widely used when faced against implementing an interaction between classes. In this tutorial, we are only going to look at hashCode(). Method Definition and Implementation hashCode(): By default, this method returns a random integer that is unique every time. If you execute…
Continue readingWhat are unit tests? Unit tests are a method of software testing in which small components of a Java application are being tested. Its purpose is to confirm the fact that every piece of the software behaves as expected. And even if it is, you can use unit testing to determine whether another implementation would…
Continue readingWhat is profiling? Profiling examines an application and tries to locate memory or performance issues that are associated with a Java application.What it allows you to do is obtaining data about the performance, method timing, object allocation, etc. by monitoring the JVM (Java Virtual Machine). You can use the IDE to profile theses types of…
Continue readingJava ArrayList class is a resizable array that implements the List interface. It permits all elements, including null and also implements all optional list operations. Most operations that can be run on ArrayList such as size, isEmpty, get, set, iterator and listIterator are all constant time. However, the add operation’s complexity is O(n) time. Compared to LinkedList, the…
Continue readingLinkedList class in Java uses a doubly linked list to store elements and it also provides a linked-list data structure. It implements List, just like ArrayList class, and Deque interfaces. Just like arrays, Linked List is a linear data structure but unlike arrays, the elements in the linked list are linked together using pointers. There…
Continue readingCollections that use a hash table for storage are usually created by the Java HashSet class. As the name suggests, HashSet implements the Set interface and it also uses a hash table which is a HashMap instance. The order of the elements in HashSet is random. The null element is permitted by this class. In…
Continue readingJava TreeSet class is a NavigableSet implementation that is based on TreeMap. The elements are either ordered by a Comparator or simply by their natural ordering. In terms of complexity, this implementation provides log(n) time cost for all basic operations like add, remove, contains. What is important to know about TreeSet in Java TreeSet implements the…
Continue readingLinkedHashSet class in Java differs from HashSet as its implementation maintains a doubly-linked list across all elements. This linked list defines the iteration ordering which is the order in which the elements were inserted into the set. It is called an insertion-order. If an element is re-inserted into the set, the insertion order is not affected by…
Continue readingJava EnumSet class implements Set and uses it with enum types. EnumSet (as the name suggests) can contain only enum values and all the values belong to the same enum. In addition, EnumSet does not permit null values which means it throws a NullPointerException in attempt to add null values. It is not thread-safe which means if required,…
Continue readingJava 8 finally allows us, the programmers, to create thread-safe ConcurrentHashSet in Java. Before then, it was simply not possible. There were variations that tried to improvise the implementation of the above-mentioned class, one of which would be, using ConcurrentHashMap with a dummy value. However, as you might have guessed, all of the improvisations of…
Continue readingArrays’ items are stored as an ordered collection and we can access them by indices. HashMap class in Java on the other hand, stores items in a group pairs, key/value. They can be accessed by an index of another type. This class does not guarantee that there will be a constant order over time. HashMap provides…
Continue readingLinkedHashMap is a combination of hash table and linked list that implement the Map interface with predictable iteration order. The difference between HashMap and LinkedHashMap is that LinkedHashMap maintains a doubly-linked list which allows scanning through all of its entries back and forth. The order is maintained, meaning the order in which keys were inserted…
Continue readingTreeMap implements the Map interface and also NavigableMap along with the Abstract Class. The map is sorted according to the natural ordering of its keys or by a Comparator provided a the time of initialization. In terms of time complexity, this implementation provides log(n) cost for the containsKey, get, put and remove operations. It’s important…
Continue readingEnumMap class implements the Map class and enables the use of enum type keys. Enum maps are maintained in the natural order of their keys. It is important to note that null keys are not permitted. If attempt has been made for adding a null key, NullPointerException will be thrown. However, even though null keys are not allowed, null values are. Since all…
Continue readingWeakHashMap in Java implements the Map interface and represents a hash table that has weak keys. If a key is not in an ordinary use, the entry from the map will be automatically removed. This is what differentiates it from other Map implementations. Null and non-null values are supported and the performance is similar to HashMap class in and…
Continue readingIdentityHashMap implements the Map interface and two keys are considered equal when checked as k1==k2 (not by using equals method). This itself violates the Map‘s general contract, which means that IdentityHashMap is clearly not a general-purpose Map implementation. There are only so many cases where this class would be useful. IdentityHashMap permits both null values and the null key, alongside all optional map…
Continue readingSortedMap interface extends Map and ensures that all entries are in an ascending key order (hence SortedMap). If you want to have it in a descending order, you will need to override the Compare method in the SortedMap which we will do shortly. TreeMap implements SortedMap and it either orders the keys by their natural…
Continue readingEven though Map is implemented by many classes, many of them are not thread-safe or some of them are but not efficient. This is why ConcurrentMap was introduced in Java 1.5. It is thread-safe and efficient. Overridden default implementations: compute replaceAll forEach getOrDefault computerIfAbsent computerIfPresent ConcurrentMap consists of an array of nodes that are represented as table…
Continue readingHashtable implements a hash table (as the name suggests) and maps keys to values (like LinkedHashMap). Hashtable class allows non-null objects to be used as a key or as a value. Just like HashMap, Hashtable has two parameters that affect its performance: initial capacity and load factor. The capacity is the number of buckets in the hash…
Continue readingGraphs are usually made from vertices and arcs. Sometimes they are also called nodes (instead of vertices) and edges (instead of arcs). For the sake of this tutorial I will be using nodes and edges as reference. Graphs usually look something like this: In many cases, the nodes and the edges are assigned values to…
Continue readingSearching and/or traversing are equally important when it comes to accessing data from a given data structure in Java. Graphs and Trees are an example of data structures which can be searched and/or traversed using different methods. Depth-first-search, DFS in short, starts with an unvisited node and starts selecting an adjacent node until there is…
Continue readingSearching or traversing is really important when it comes to accessing data from a given data structure. There are different methods of traversing/searching elements within these data structures such as Graphs and Trees. Breadth-first search is one example of these methods. BFS is an algorithm that traverses tree or graph and it starts from the…
Continue readingBefore starting to explain the different time complexities, let’s first look at what an actual algorithm is. The formal definition of an algorithm is “a process or set of rules to be followed in calculations or other problem-solving operations, especially by a computer.”. So in other words, an algorithm is a defined path which is…
Continue readingSerialized object. What does that mean? Java provides a functionality which represents an object as a sequence of bytes which include the object’s data and also information about the object’s type and the types of data stored in that object. When a serialized object has been written into a file, it can later be deserialized,…
Continue readingReflection (which is a feature in Java) allows executing Java program to examine itself (or another code) and manipulate internal properties of the program such as obtaining names of members and perform something on them, like deleting them or displaying them. By default, every object in Java has getClass() which basically determines the current object’s…
Continue readingJava 8 introduced a new Date-Time API which purpose is to cover drawbacks of the old date-time API. The previous date-time api was not thread safe and the replacement for the new date-time API is that it does not have any setter methods. Another drawback that is fixed by the new API is the poor…
Continue readingThe JavaMail API “pp”-independent(platform and protocol) framework which purpose is to help build messaging and mail applications. It is
Continue readingIn short, Docker is a tool that allows you to build, deploy and run applications easily by the usage of so-called containers. These containers let us package all the essentials such as libraries and dependencies. In addition, the containers run on the host operation system. There are many benefits that come when we use Docker. It…
Continue readingControllers’ main purposes in Spring are intercepting incoming http requests, sends data to Model for processing and finally gets processed data from the Model and passes the very same data to View which is going to render it. A very top-level overview of the written above: Now, let’s build a simple app which will serve…
Continue readingJust like @RequestParam, @PathVariable annotation is used to extract data from HTTP request . However, they differ slightly. The difference is that @RequestParam gets parameters from the URL while @PathVariable simply extracts them from the URI. Example Let’s imagine you had a website that supported the following URL: http://www.yourwebsite.net/employee/1 1 in the URL above represents…
Continue readingThe @RequestBody annotation can be used for handling web requests. More specifically, it is used to bind a method parameter with the body of a request and the way it works is HttpMessageConverter converts the request’s body based on the type of the content of the request. Syntax <modifier> <return-type> <method-name> (@RequestBody <type> <name>) { }…
Continue readingThe RequestParam annotation is used when we want to read web request parameters in our controller class. In other words, the front end is sending us some parameters (from a filled form for example) with keys. Use case Suppose we had a form which purpose was to add an employee to the database. Each employee would have:…
Continue readingIn Spring, Interceptors, as the name suggests, intercept we requests through implementing HandlerInterceptor interface. It provides us with methods that allow us to intercept incoming requests that is getting processed by the controller class or the response that has been processed by the controller class. The methods that the interface provides us with are: preHandle() – returns true…
Continue readingA famous example of explaining the Inversion of Control (IOC) concept is the Holywood principle which states “Dont’ call us, we will call you”. Without a doubt, it is a very accurate analogy, hence the amount of people referencing it as an analogy. Usually, classes create dependencies. However, IOC gives us the opposite functionality –…
Continue readingIn a nutshell, the IoC container is responsible for instantiating/creating and configuring an object and assembling the dependencies between objects. You might be wondering.. how does IoC container receive the data to do the above-mentioned? The answer is from 1 of 3 places: XML fle, Java code or Java annotations. IoC Container is a framework…
Continue readingThe dispatcher servlet is the most important component in the Spring Web MVC. Why is the dispatcher servlet the most important component though? Because it acts as a glue, meaning it receives an incoming URL and finds the correct methods and views. It receives the URL via HTTP request. You can also think of it…
Continue readingIn this tutorial you are going to learn what Dependency Injection in Spring is, how it works and how you can use it. What is Dependency Injection? Dependency Injection is one of the fundamentals of Spring which you must know. When you create a complex application, chances are you are going to have different objects…
Continue readingIn this tutorial, you are going to learn what Spring ORM is and how to use it. What is Spring ORM? Spring ORM is covers many technologies like Hibernate, iBatis and JPA. Spring provides integration classes thanks to which, each of the technologies mentioned are able to be implemented following the Spring principles of confiugration. The…
Continue readingBefore I jump into implementing the classes, let’s first understand what DAO is. If you already know what DAO is, feel free to jump to the code examples. If not, bear with me. DAO stands for Data Access Object and it is a structural pattern which isolates the business layer (logic) from the persistence layer…
Continue readingIn this tutorial you are going to learn how to create unit tests for DAOs. As a prerequisite, you fundamental knowledge of DAOs is expected. When it comes to testing DAO components, we really have 2 approaches. One is to use the mocking framework Mockito and the other is to create a couple of classes…
Continue readingAs you may be well aware, testing is very important. In this tutorial therefore, you will learn how to do just that! Testing. And more specifically, you will learn how to perform that testing on Controllers and Services. Controllers Normal controller does 1 of 2 things: renders a view or handles form submission Let’s look at…
Continue readingIn the end of this tutorial, you will have installed the right MySQL products needed to develop applications in Spring. You will need two essential things: MySQL Database and MySQL Server. Follow the steps below. Configuring the MySQL Database Visit https://www.mysql.com/downloads/ and then select Community downloads, like so: On the next page, you will see…
Continue readingIn this article, you are going to learn how to use Spring Security to achieve a login authentication functionality. The login page represents a form which asks for details such as username and password. That same login page can be done in Angular and the authentication process itself will be performed by Spring Security. There…
Continue readingIn this tutorial you are going to learn how to secure your web application using the Spring Security including user authentication, authorization and more. Spring Security – Introduction Spring Security is a customizable authentication framework. It is the standard when it comes to securing Spring-based applications. It provides both authentication and authorization. Authorization is also…
Continue readingWhen we are dealing with RESTful web services, we need to be using @RestController annotation which basically represents the @Controller and @ResponseBody annotations. When we use @RequestMapping for our methods, we can add an attribute which is called produces which specifies that the output sent to the user will be in JSON format. Example Employee.java public class…
Continue readingIn this tutorial you will learn how to protect your application against CSRF. What is CSRF? If you already know what CSRF, feel free to continue without reading this sub-point. But if you don’t, CSRF stands for cross-site request forgery and simply put, it is when attackers make authenticated users to perform an action on the…
Continue readingOAuth2 allows third-party applications to receive a limited access to an HTTP service which is either on behalf of a resource owner or by allowing a third-party application obtain access on its own behalf. Thanks to OAuth2, service providers and consumer applications can interact with each other in a secury way. Workflow There are a…
Continue readingIn this tutorial you are going to learn what Spring Boot is and how you can start using it. Prerequisites 10-20 minutes of your time familiarity with Maven What is Spring Boot? Spring Boot makes the process of creating a stand-alone Spring based application very easy and simple. By using the Spring Boot Java-based framework,…
Continue readingMVC stands for Model-View-Controller and Spring supports it. The great thing about the MVC pattern is that it separates different aspects of the application like inputs, business logic and user interface. This pattern is so widely used that it even has a song about it. The three components in the MVC pattern are Model, View, Controller,…
Continue readingIn this tutorial you are going to learn what the JDBC module is and hopefully you will be able to find use cases after you are done reading it. Now, let’s create a very simple table that represents an employee. CREATE TABLE Employee ( ID INT NOT NULL AUTO_INCREMENT, NAME VARCHAR(20) NOT NULL, AGE INT…
Continue readingIn this tutorial, you are going to learn what is Docker and how we can use it to Dockerize a Spring application. Dockerfile Dockerfile is just a .txt file. Dockerfile allows you to run commands that help you build an image. These commands can be useful if you want to specify the layers of the image. One…
Continue reading@Autowired annotation is a relatively new style of implementing a Dependency Injection. It allows you to inject other beans into another desired bean. Similar to the @Required annotation, the @Autowired annotation can be used to “autowire” bean on setter methods as well as constructors and properties.. @Autowired Annotation on Setter Methods Please note that when…
Continue readingIf you are familiar with Spring, you’ve probably heard of Aspect Oriented Programming (AOP). That’s one of the main components of the Spring framework. However, no previous experience in AOP is needed. It is focused for complete beginners who want to understand how AOP framework in Spring works. In Object Oriented Programming, modularity of an…
Continue readingIn this tutorial you are going to learn what Sping Bean is and how to use it. What is Spring Bean? Beans are the objects that construct the application and are managed by the Spring IoC container. The formal definition from the Spring Framework documentation is: In Spring, the objects that form the backbone of…
Continue readingBefore testing the MySQL connection from a Java program, we’ll need to add the MySQL JDBC library to the classpath. We will need to download the mysql-connector-java-*.jar file from the downloads page: Now, depending upon your work environment (e.g. Eclipse or Command line) you will have to do either one: If working with Eclipse IDE,…
Continue readingDebugging – the technique one uses most and is inevitable. If only there was a tool that allowed us to make this sometimes-tedious task much easier and not-so-tedious… oh wait. There is. Eclipse allows to start a Java programin in the so-called Debug mode. What it is most useful for is that it allows you…
Continue reading